Ultrasonic Parking Assistant
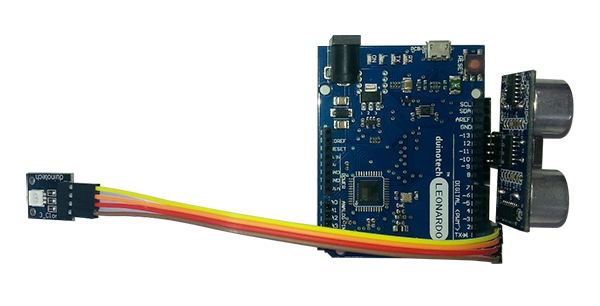
Summary
We saw a version of this project in The Shed Magazine, and thought it would be perfect to build using our duinotech parts. It simply uses an ultrasonic distance sensor to measure how far away the car is from the sensor, and then light up an RGB LED module to let you know how close you are. A simple job perfectly suited to an Arduino.
Table of Contents
Future Improvements
To make full use of the Ultrasonic Parking Assistant, it should be mounted on something fixed and pointing towards where the car will be approaching (ideally, right towards the number plate). We found that it could be made flat by gently bending the pins on the ultrasonic sensor and jumper leads 90 degrees, like below:
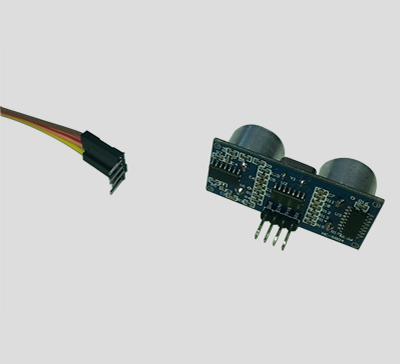
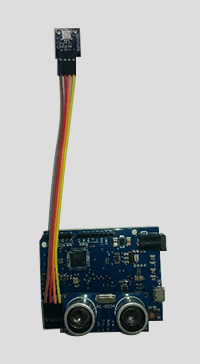
Be careful bending the pins, as they can snap off if bent too far. After this, it could simply be attached to a wall using double-sided tape, being careful to ensure the bare pins don’t short out on anything metal. The next step is to power it. You could use a 12V Plug Pack, or look at other power ideas for Arduino projects in our Powering Your Arduino guide.
None of the duinotech parts are waterproof, so we recommend that it not be installed outdoors.
Apart from these ideas, you could add a Buzzer Module to give an audible warning as well as visual- this would need to added to the code though. If the LED isn't visible, multiple jumper leads can be connected end to end to extend it further, and the LED can of course be attached to a wall with some of the double sided tape.
Similar projects you may be interested in